Build a Slack chatbot in Laravel with BotMan Studio 2.0
#chatbotsWith BotMan it is really easy to setup a Slack chatbot. Last week the new 2.0 version was released. We will checkout how to setup a Slack chatbot in this new version with BotMan Studio step by step.
Preparations
Before we start, make sure to have these things prepared:
- PHP7+ environment
- ngrok or Laravel Valet to get a public URL to your BotMan app
- A Slack account and team
Install BotMan Studio
The easiest way to install BotMan Studio is via the installer.
composer global require "botman/installer"
After that you can just install a new instance like that:
botman new botman-Slack
It is basically like the Laravel Installer. Your application is now already installed. When you use Laravel Valet you can directly check the homepage, botman-slack.dev
in my case. Here you will see the BotMan Studio welcome page.
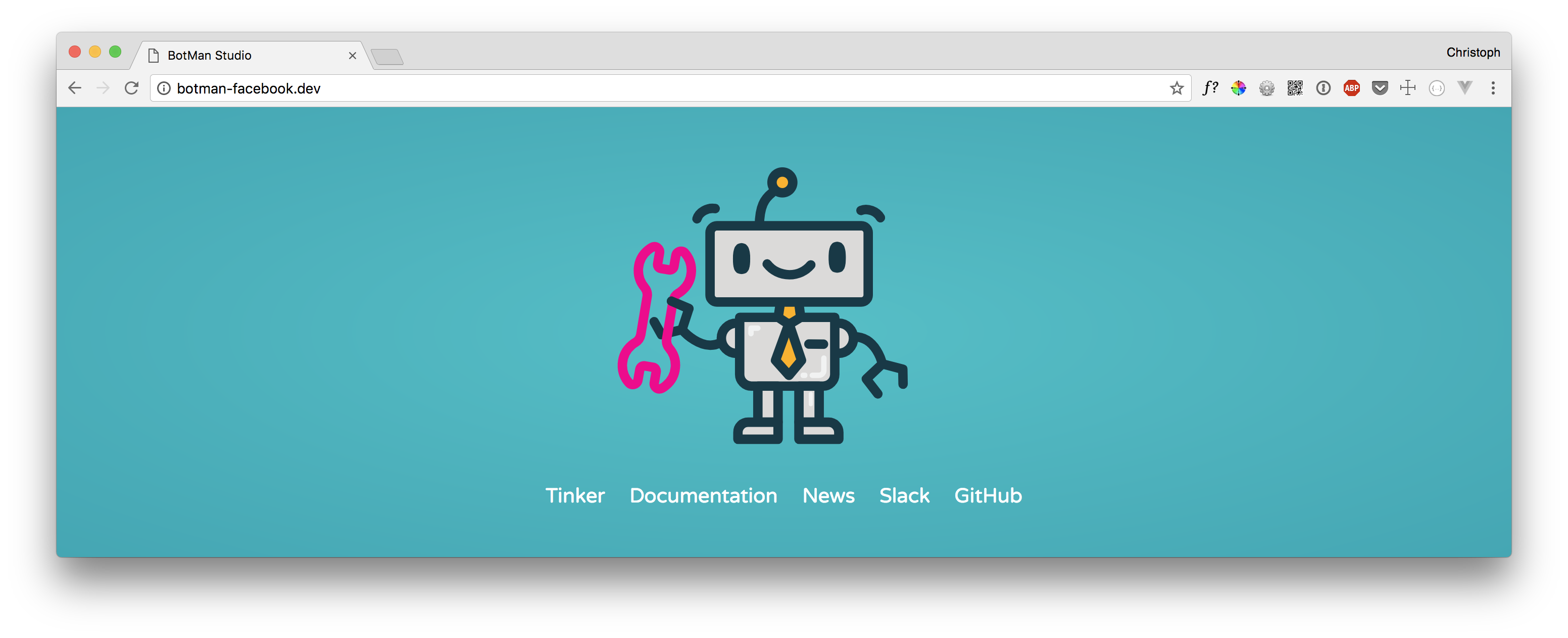
When you click Tinker
you can immediately test your chatbot. Type Hi
and you should get a reply. This works because this behaviour comes with BotMan Studio. You will find the code for that in your routes/botman.php
file.
$botman->hears('Hi', function ($bot) {
$bot->reply('Hello!');
});
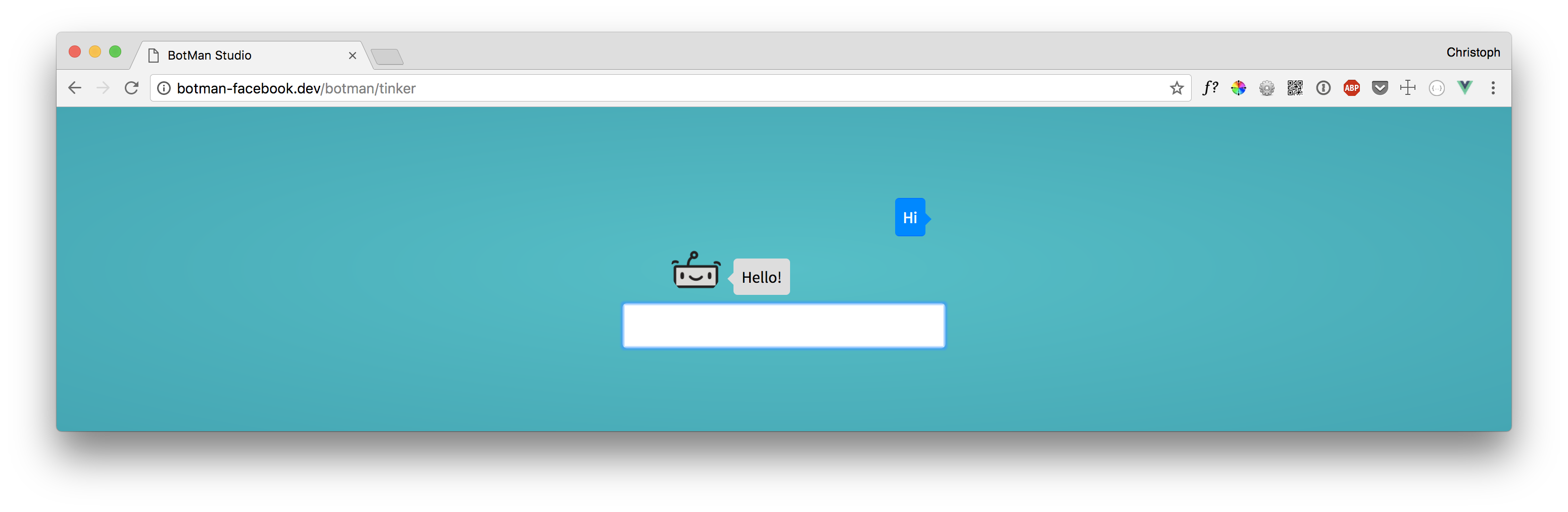
Configure BotMan Studio
Now that BotMan is installed we need to configure it to work with Slack. When you use the BotMan artisan command php artisan botman:list-drivers
you will see the installed driver.
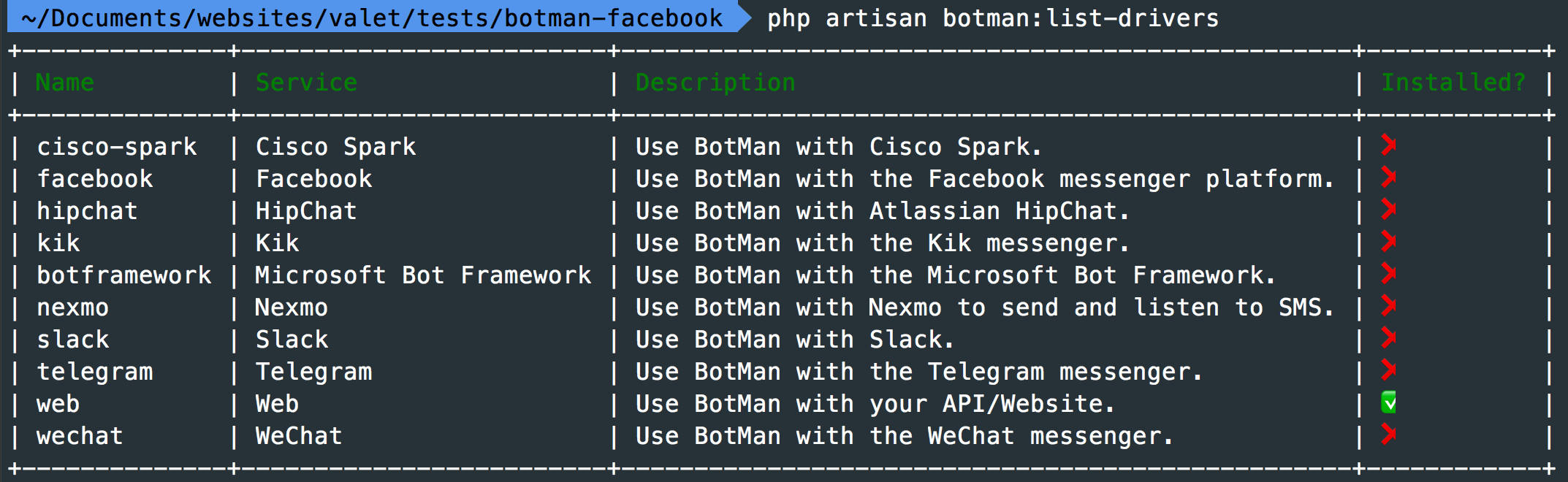
As you can see, by default only the web driver is installed. Since version 2.0 almost all drivers live in separate GitHub repositories and you need to install the ones you need. In order to install the Slack driver we can use another artisan command.
php artisan botman:install-driver slack
Next to the driver this will also add a config/botman/Slack.php
config file. There you'll see that BotMan requires some data from your .env
file.
<?php
return [
/*
|--------------------------------------------------------------------------
| Slack token
|--------------------------------------------------------------------------
|
| Your Slack bot token.
|
*/
'token' => env('SLACK_TOKEN'),
];
In order to connect our BotMan application to Slack, we need the Slack token. We will receive that later.
Create a Slack app
There are multiple ways to use BotMan with your Slack team. The recommended one is by using a Slack app. This is why I will only cover this one here. Read the official docs for more information about the other possibilities.
Visit the Slack API website in order to create a new Slack app.
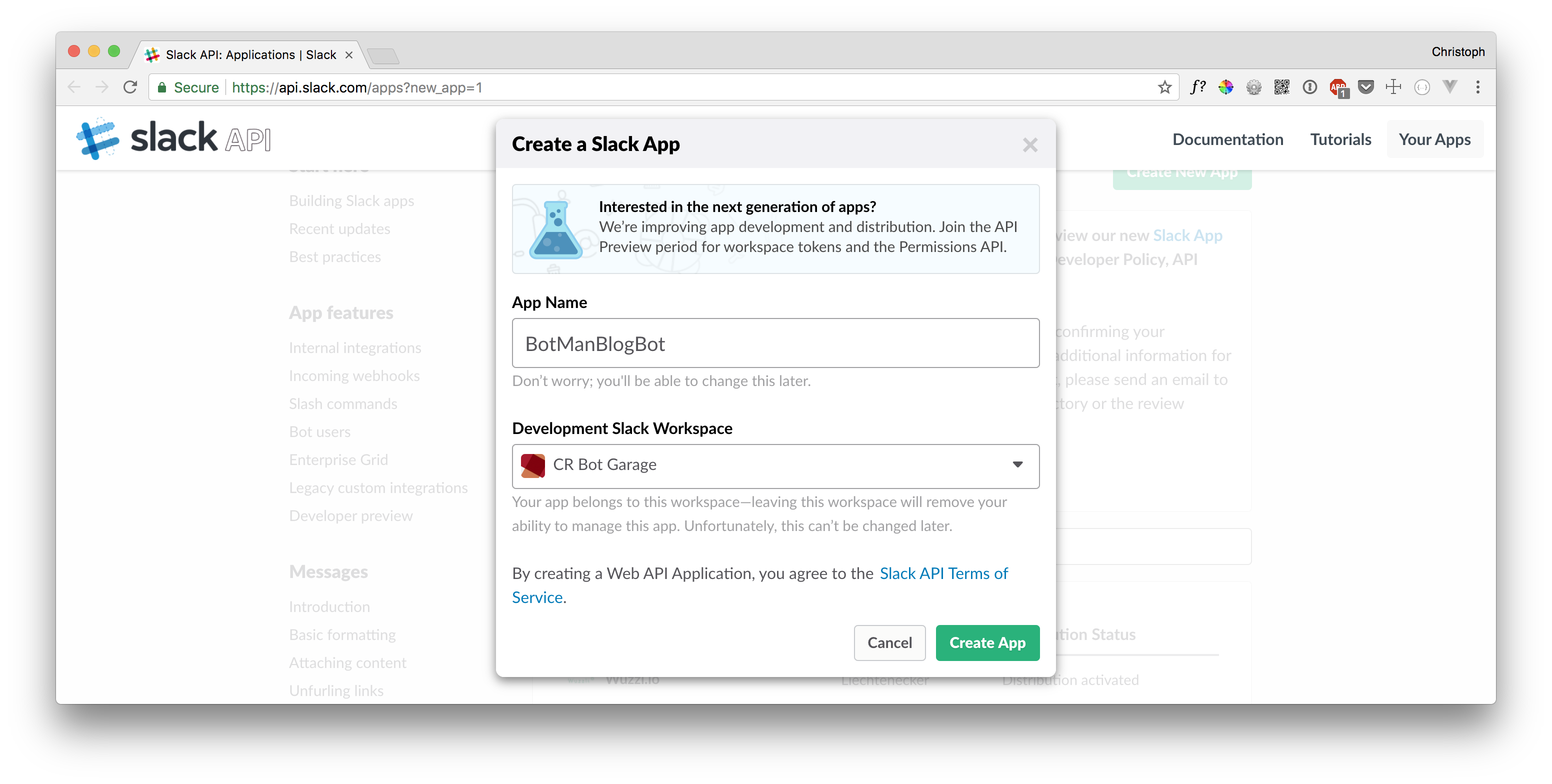
Once we have an app we can configure it. Here is a list of what we want to do:
- Active Interactive Messages
- Define Events to listen to
- Setup the Bot User
- Install the app
Active Interactive Messages
When you use buttons with your Slack messages, they will trigger Interactive Messages that will be sent to BotMan. So we need to active them and place our BotMan webhook URL in the Request URL
field.
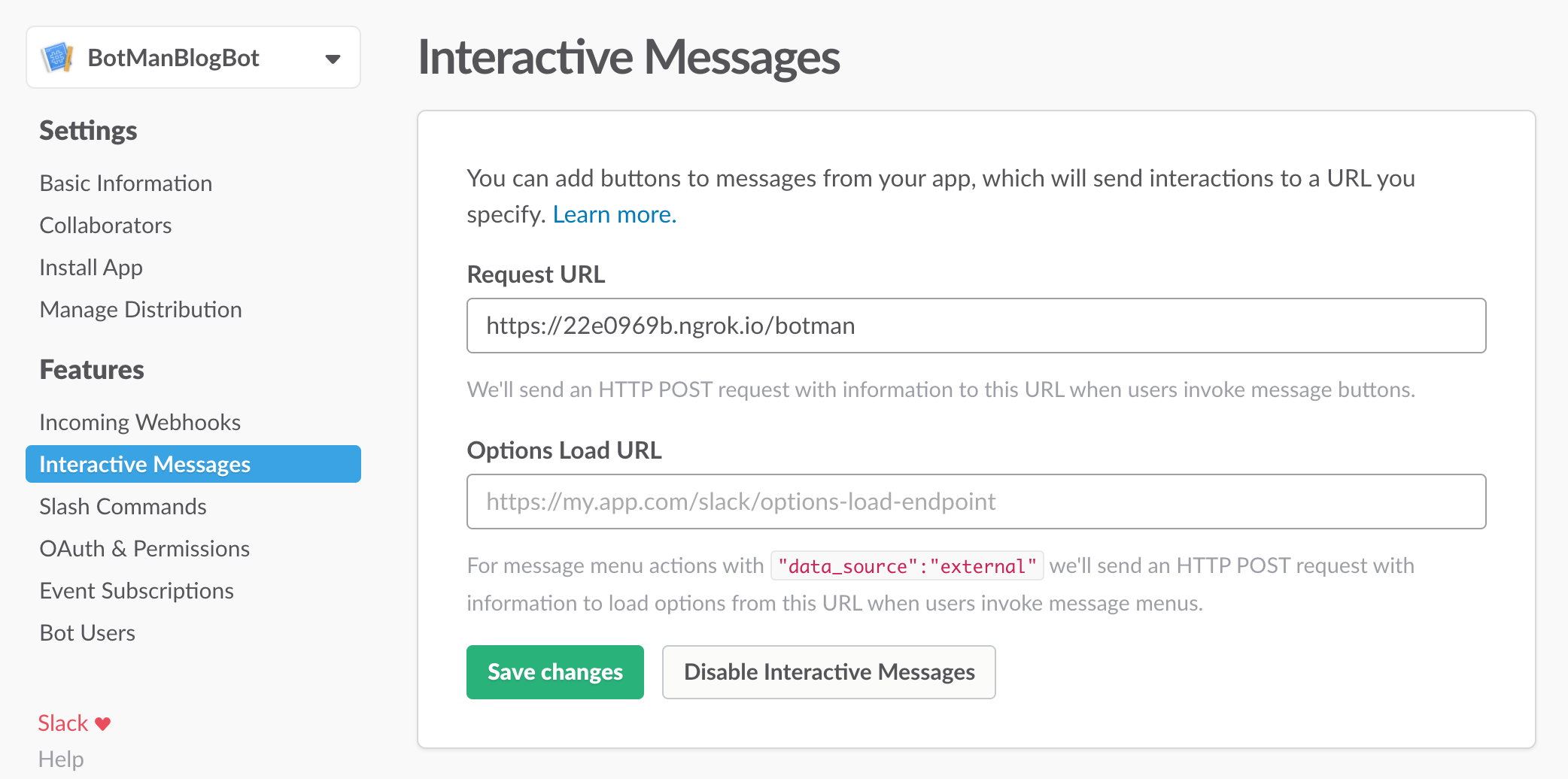
Define Events to listen to
In order to listen to Slack messages we need to subscribe to those events. Only then we are able to receive the messages and reply to them. Here we are saying that we want to get all messages from channels and direct messages. Again we need to fill in our BotMan webhook.
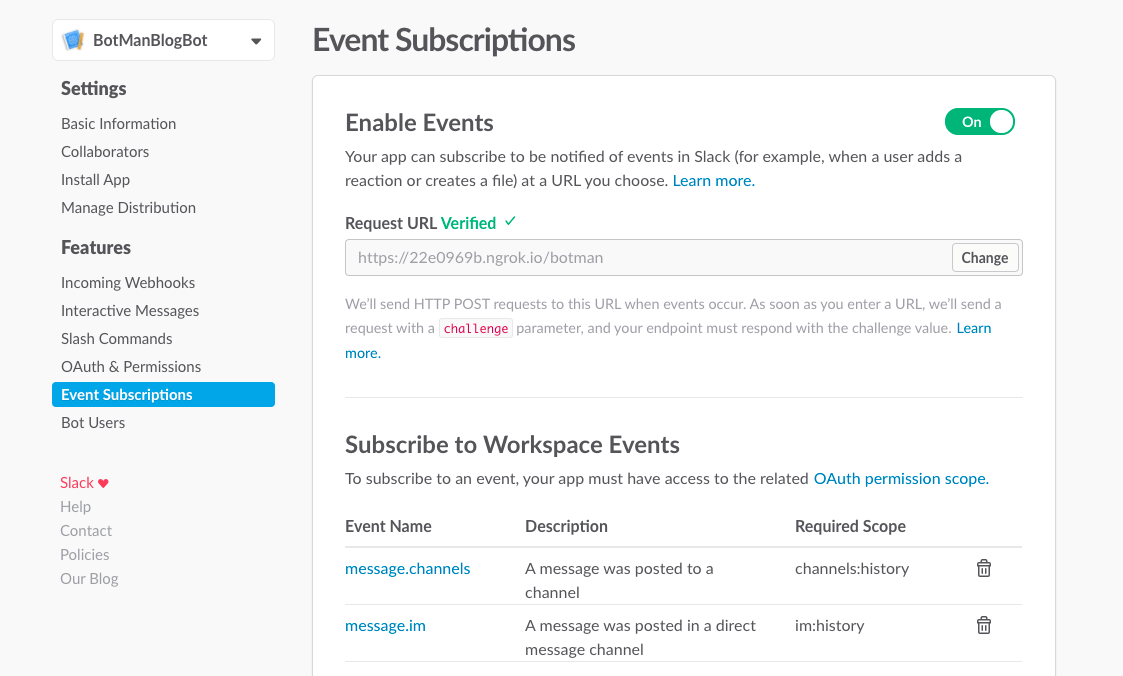
Setup the Bot User
Right now we just have a configured app. But what we want is a "real" user in our Slack Team. This is what the Bot Users
section is for. Just give your bot a display and username.
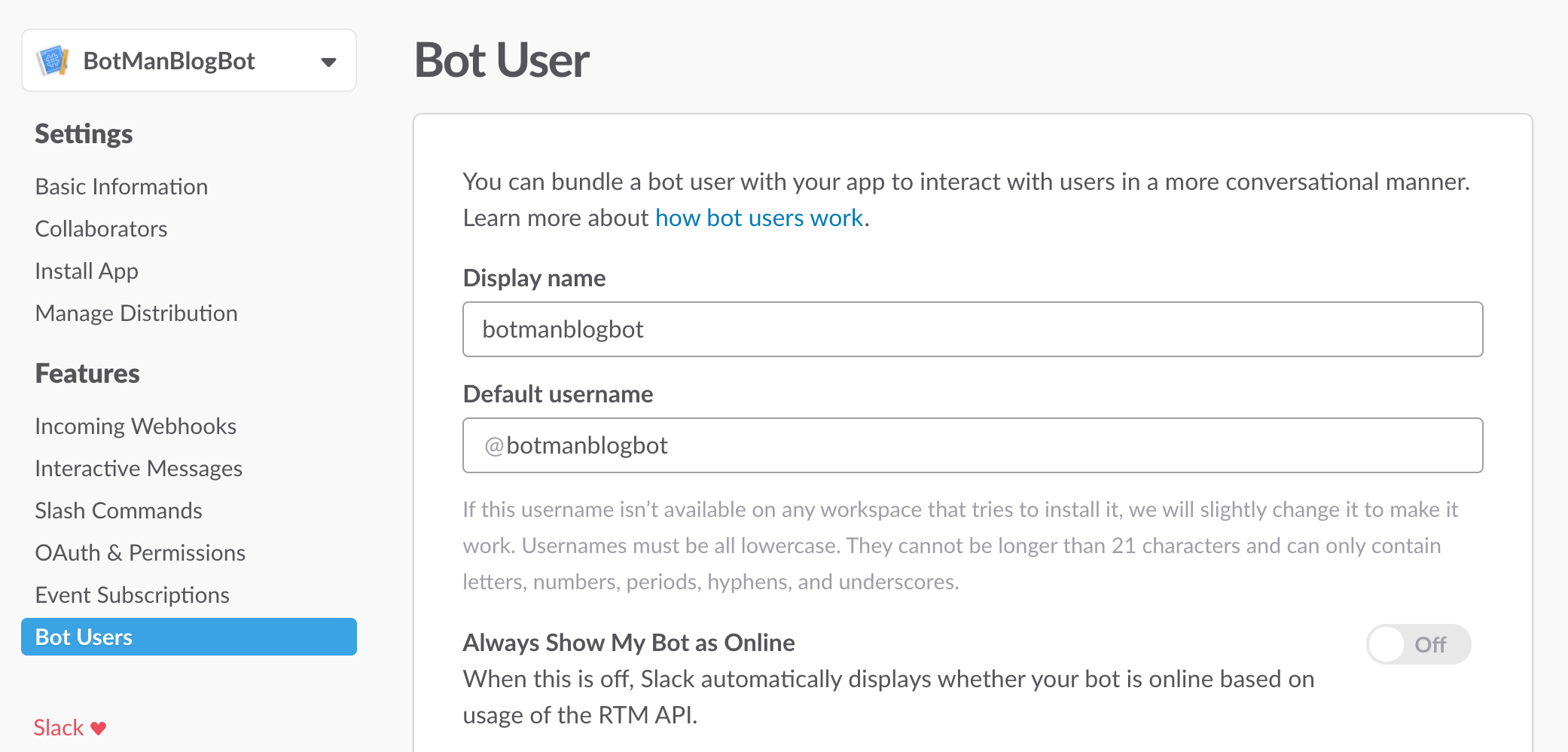
Install the app
Now we're almost finished. Last step is to install
the app. Just hit the button and you will get the tokens.
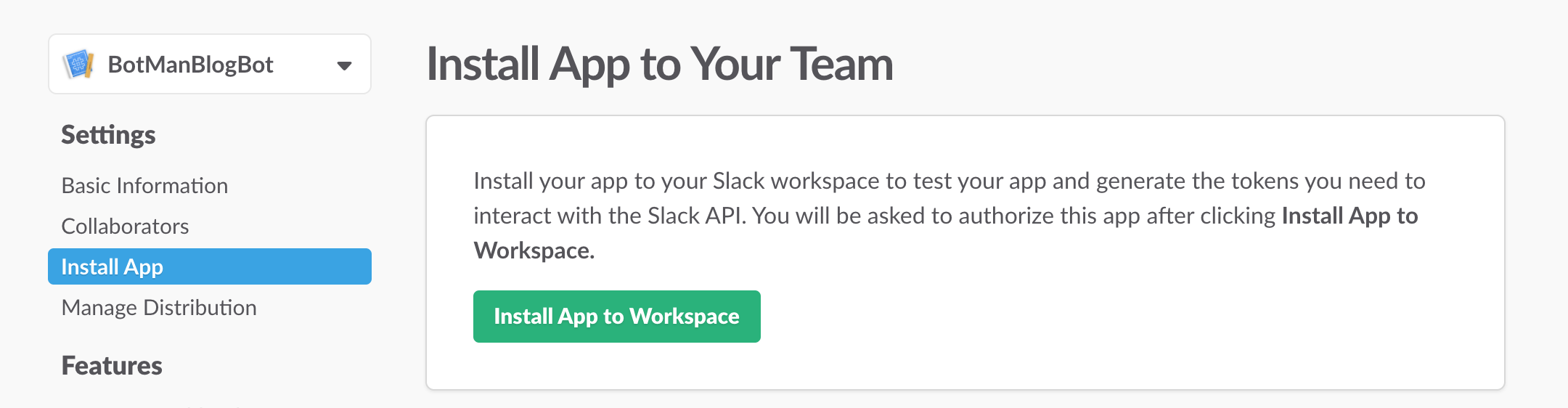
The Bot User OAuth Access Token
is the one that we need. Place in your .env file, because your slack.php
config file is loading it from there.
SLACK_TOKEN=YOUR_SLACK_BOT_USER_TOKEN
Test it out
Next we can test if everything is working like planned. So visit your Slack Team and you should see the new Slack user there. Just send a message with Hi
. You should again get a reply with Hello!
.
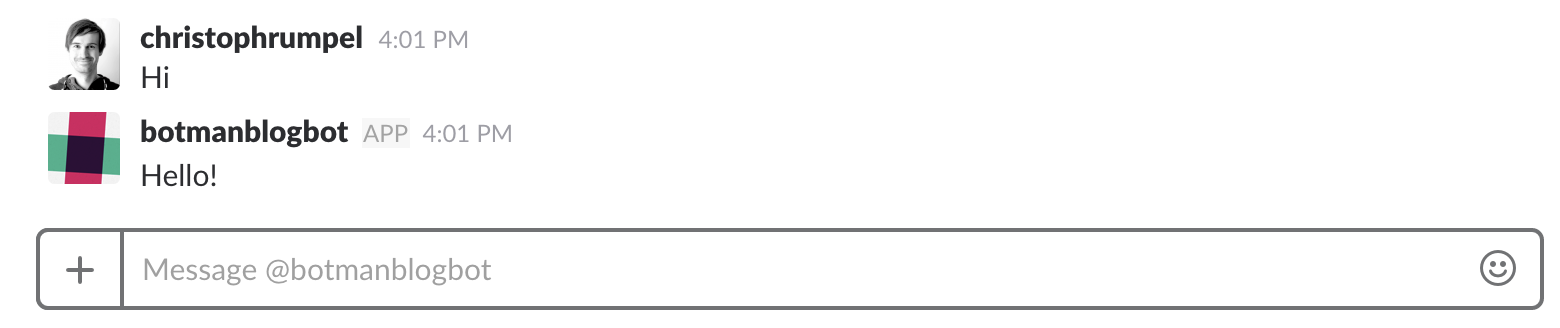
Additionally we can test the example conversation, which is built into BotMan Studio.
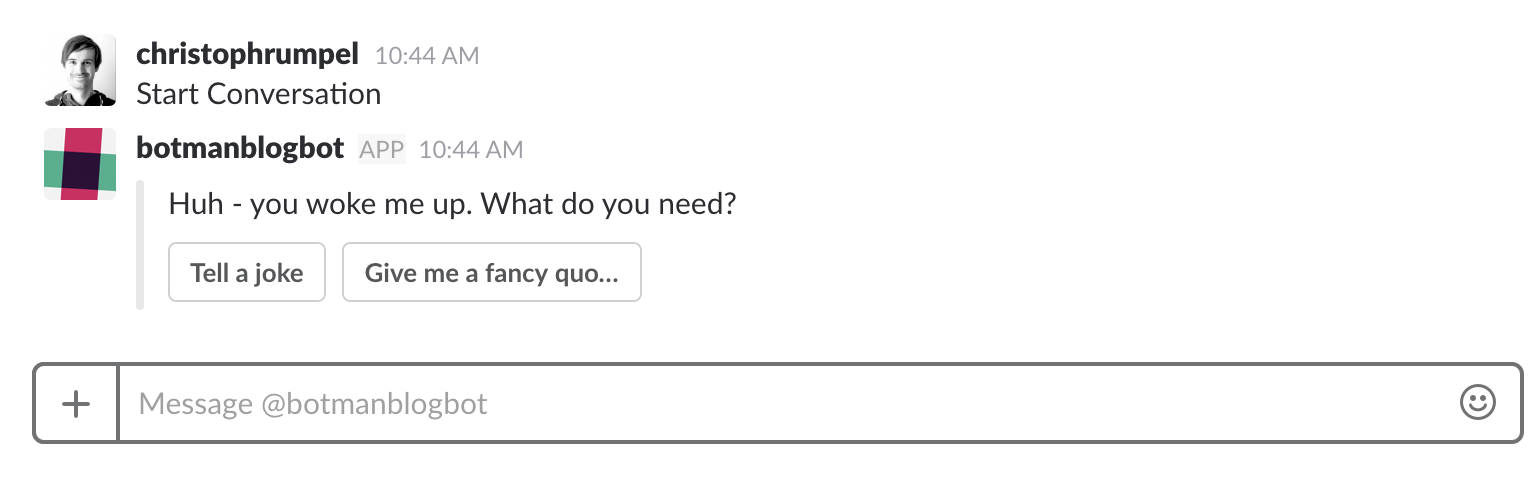
First custom message
And in order to write some chatbot functionality ourselves, we add a custom listener to the routes/botman.php
file.
$botman->hears('It just works', function(BotMan $botMan) {
$botMan->reply('Yep 🤘');
});
You will see that this works like a charm as well.
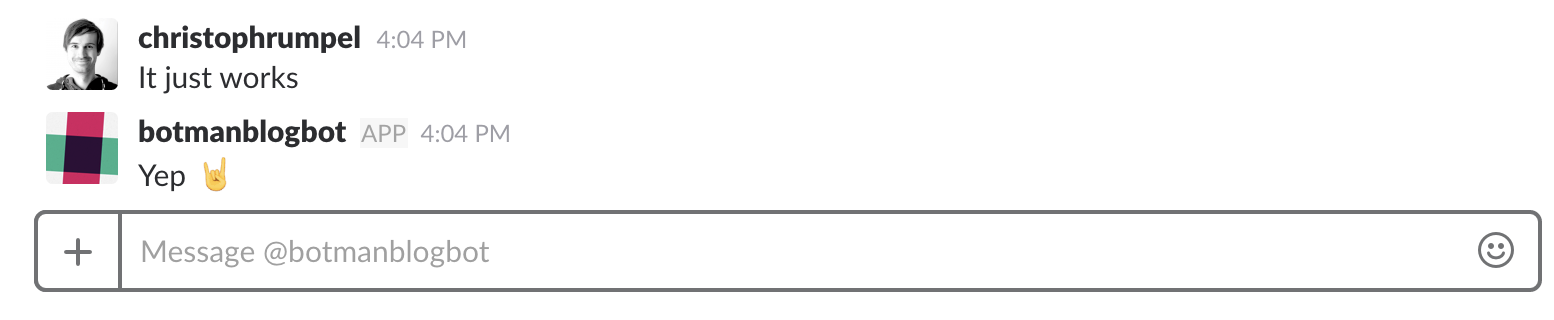
Conclusion
As you've seen, most of the things we need to do is on the Slack site. Once that is done BotMan just works and is easy to extend. I hope I could help you with your first steps and that this article helps you to setup your next Slack bots. From here you are ready to build more and more features to your bot your own. So make sure to checkout the BotMan documentation to get a feeling of what is possible and learn new stuff.