Using Facebook Messenger Quick Replies with BotMan
#chatbots,botmanI guess you already used quick replies for text buttons, right? But did you know that you can ask the user for email, phone number, and current location? It is time to give you a refresh of Facebook Messenger quick replies and how they work in the BotMan chatbot framework.
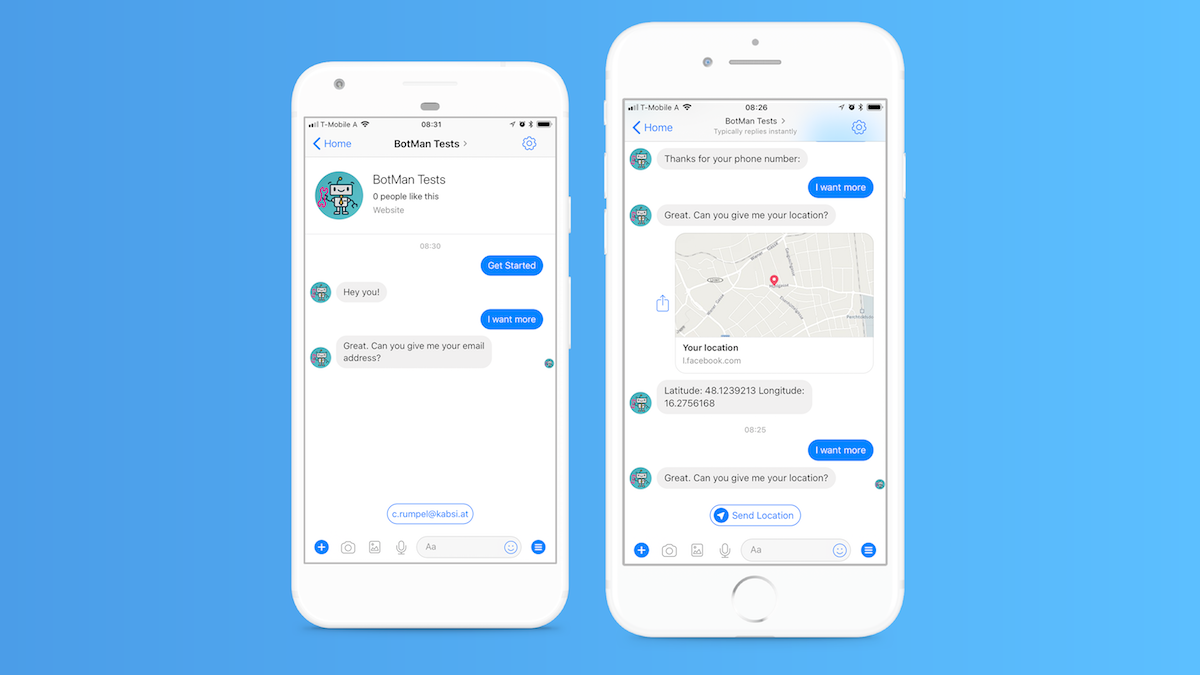
Within chatbot conversation, it is a good idea to provide users with possible reply options. In Facebook Messenger, these options are called quick replies
. Quick replies are buttons that the user can click instead of writing a reply himself. After clicking, the other options will disappear and only the clicked button text, will stay in the chatbot as the user's reply.
Structured messages, like quick replies, help users orientate within a conversation and make them simpler and faster. There are lots of other types of structured messages in Facebook Messenger, but today we will concentrate on quick replies.
Prefer the video?
Sending Quick Replies
In BotMan it is effortless to send quick replies. The default BotMan question object with buttons will result in quick reply options. This is how you can send them from within your botman route file.
$botman->hears('I want more', function (BotMan $bot) {
$bot->reply(Question::create('Are you sure?')->addButtons([
Button::create('Yes')->value('yes'),
Button::create('No')->value('no'),
]));
});
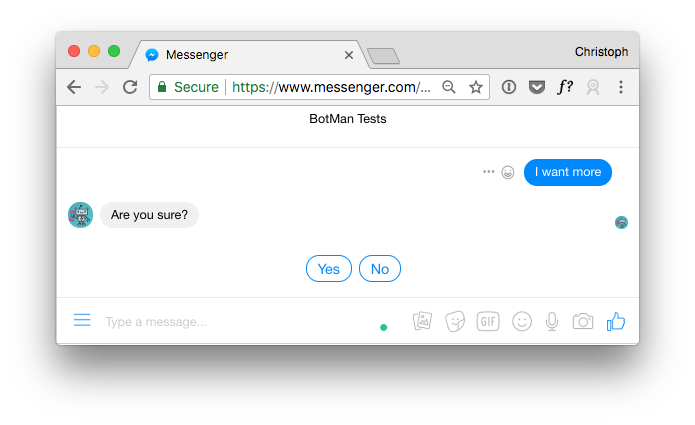
Listening To Quick Replies
BotMan makes it easy for you to handle clicked buttons. You can listen to their value
. Here is another example.
$botman->hears('I want more', function (BotMan $bot) {
$bot->reply(Question::create('Are you sure?')->addButtons([
Button::create('Yes')->value('yes'),
Button::create('No')->value('no'),
]));
});
$botman->hears('yes', function (BotMan $bot) {
$bot->reply('Awesome 🤘');
});
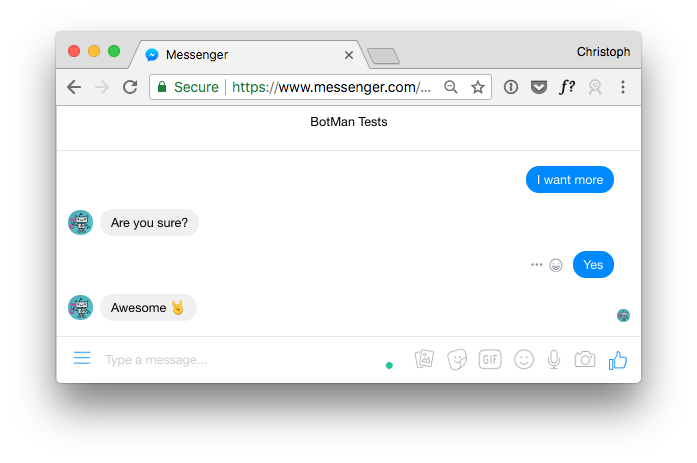
Using Quick Replies In Conversations
Most of the times you ask the user something, it will be part of a conversation. This is when it is a better idea to use a conversation class instead of listening in your BotMan route file for replies. First, we create a conversation class.
php artisan botman:make:conversation QuickReplyConversation
We will listen again for the sentence "I want more", but now we start a conversation to handle everything else. Don't forget to import the conversation class namespace as well.
$botman->hears('I want more', function (BotMan $bot) {
$bot->startConversation(new QuickReplyConversation());
});
Then we ask our question and provide options for the replies.
<?php
namespace App\Conversations;
use BotMan\BotMan\Messages\Incoming\Answer;
use BotMan\BotMan\Messages\Outgoing\Question;
use BotMan\BotMan\Messages\Outgoing\Actions\Button;
use BotMan\BotMan\Messages\Conversations\Conversation;
class QuickReplyConversation extends Conversation
{
/**
* Start the conversation.
*
* @return mixed
*/
public function run()
{
$this->askAboutMore();
}
private function askAboutMore()
{
$question = Question::create('Are you sure?')->addButtons([
Button::create('Yes')->value('yes'),
Button::create('No')->value('no'),
]);
$this->ask($question, function (Answer $answer) {
if($answer->getValue() === 'yes') {
$this->bot->reply('Awesome 🤘');
}
});
}
}
Ask For Location, Phone number, and Email with Quick Replies
Since Messenger Platform 2.3, quick replies also support location
, phone number
and email
. This means that the chatbot can ask for one of these values from the user. Facebook has access to these values and can send them to the bot if the user wants to.
Let's start with the email address. Instead of using the default Button
class, we switch to the QuickReplyButton
. On its instance, we can then set a type, user_email
in this case.
$question = Question::create('Great. Can you give me your email address?')
->addAction(QuickReplyButton::create('test')->type('user_email'));
$this->ask($question, function (Answer $answer) {
$this->bot->reply('Thanks for your email: '. $answer->getValue());
});
This will result in this button with the user's email address. Through clicking it, the user sends it to the chatbot. You can then access the email address with $answer->getValue()
.
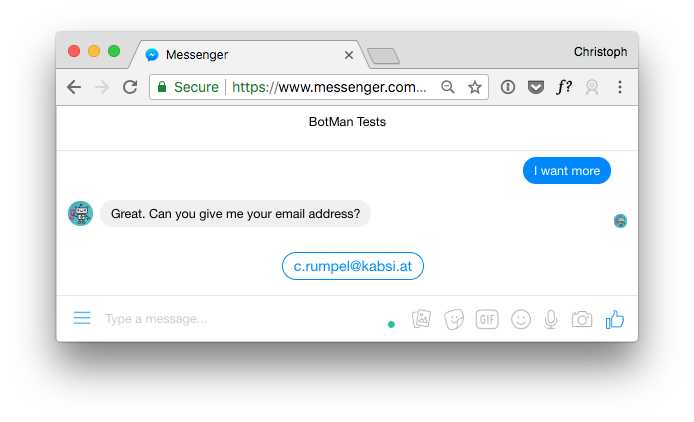
Phone
Asking for the phone number is very similar to the email quick reply. Only the type is changing.
$question = Question::create('Great. Can you give me your phone number?')
->addAction(QuickReplyButton::create('test')->type('user_phone_number'));
$this->ask($question, function (Answer $answer) {
$this->bot->reply('Thanks for your phone number: '. $answer->getValue());
});
Since I don't have my phone number connected to my Facebook account, I can't show you a screenshot of that. Important to know is, that if you don't have a phone number connected, the quick reply button will not be shown.
Location
Since the last Facebok Messenger Platform update, we are also able to ask for the user's location. This is great when you need to provide information based on where the user is right now. Again, it is very similar to the other quick replies. The only differences are the type again and that we get access through the location object after the answer.
$question = Question::create('Great. Can you give me your location?')
->addAction(QuickReplyButton::create('test')->type('location'));
$this->ask($question, function (Answer $answer) {
$this->bot->reply('Latitude: '.$answer->getMessage()->getLocation()
->getLatitude().' Longitude: '.$answer->getMessage()->getLocation()->getLongitude());
});
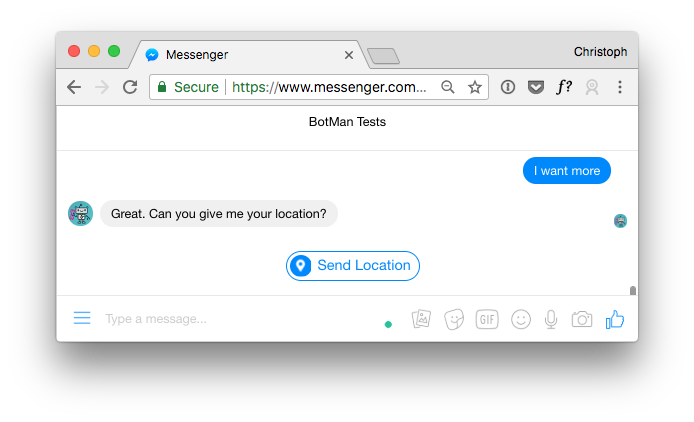
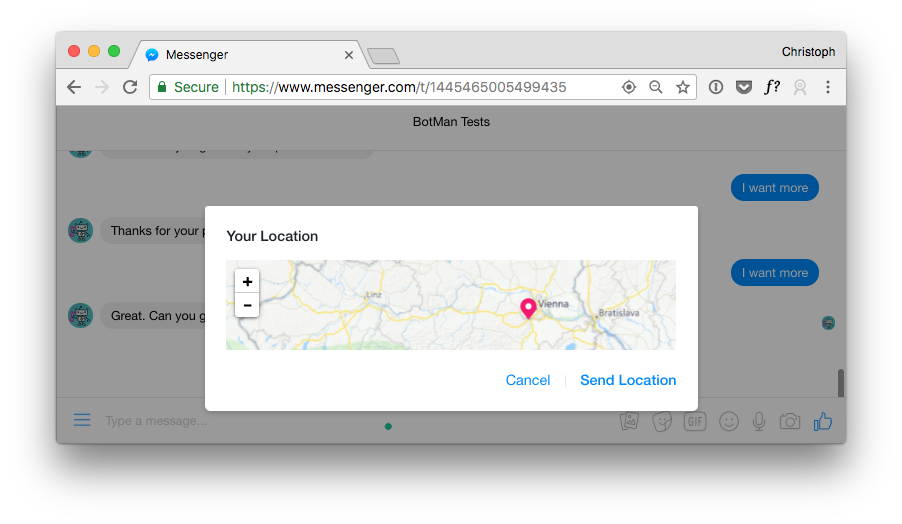
Conclusion
This was my portion of wisdom regarding Facebook Messenger quick replies. As you have seen, they help provide the user a better experience while asking for data like email, phone number or location. BotMan makes it very straightforward to use them. Time to try it out yourself!